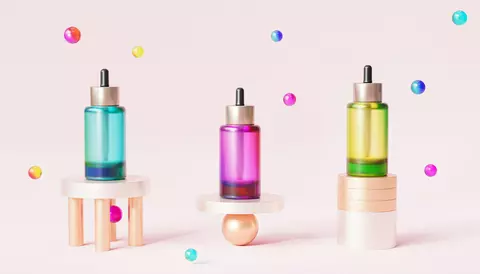
Python Flask (or Dash) applications on Plesk
Although not officially documented, it is possible to host Python Flask applications easily with Plesk. The process and technologies are the same as those used for Plesk's official Node.js support.
1. Dependencies
Plesk Extensions
In the Plesk installer, make sure Phusion Passenger server
and Nginx web server
are installed:
Server access
SSH access to the server is also required to do the initial installation of the Python packages.
Python PIP
The Python package manager (PIP) must be installed on the server. To ensure this is the case, run the following command:
python3 -m pip
If the message /usr/bin/python3: No module named pip
appears, then pip
must be installed. After logging into the server with a root user, run the following command to install it:
sudo apt-get install python3-pip
2. Adapt your Python code
When used for Python, Phusion Passenger expects to receive a Whisky App.
Most of the time when dealing with a Flask app, adding these lines will allow the app to be exposed as a Whisky app:
application = app
For a Dash application, use the following commande instead :
application = app.server
3. Deploy your Python code
Deploy your Python code to the Plesk server with the method of your choice (git, GitHub, SFTP, etc.)
For this example, we will simply create an app.py
file with Plesk's built-in file editor. The contents of this file will be as follows:
from flask import Flask
app = Flask(__name__)
@app.route("/")
def hello_world():
return "<p>Hello, World!</p>"
# The following line is required by Phusion Passenger. It exposes the WSGI App using the application variable.
# Reference : https://community.plotly.com/t/deploying-dash-app-on-a-wsgi-service/57867
application = app.server
We will also create the requirements.txt
file to indicate which Python packages are needed for the project. The contents of this file will be as follows:
Flask==2.2.2
Werkzeug==2.2.2
4. Install Python dependencies
SSH into the server to download and install the project dependencies. Once logged in, run the following commands:
cd /var/www/vhosts/flaskdemo.jmcouillard.com/httpdocs
python3.8 -m pip install -r requirements.txt
5. Test the app
At this point, your app should be available in the terminal. It is normal that it is not yet available in a web browser.
To test the application in the terminal, run this command:
python3.8 app.py
If an error is displayed, then it must be resolved before proceeding further.
6. Configure nginx
Enable nginx server without Apache
It is necessary to tell Plesk to pass requests directly to nginx, without relaying them to Apache. To achieve this, uncheck the Proxy mode option in the Apache & nginx Settings settings of your Plesk domain.
Configure nginx to use Phusion Passenger with your Python application
In the Additional nginx directives section, add the following commands, taking care to replace the values with those that apply in your project:
passenger_enabled on;
passenger_app_type wsgi;
passenger_python /usr/bin/python3.8;
passenger_startup_file app.py;
passenger_app_root /var/www/vhosts/flaskdemo.jmcouillard.com/httpdocs;
7. Automatic deployment tasks (optional)
If you chose in step 2 to deploy your code using git with a local Plesk repository, you can improve your workflow with these CI/CD optimizations.
In the repository settings, enable the Enable additional deployment actions option and add the following commands:
python3.8 -m pip install -r requirements.txt > pip-install.log 2>&1
passenger-config restart-app /var/www/vhosts/flaskdemo.jmcouillard.com/httpdocs > restart-passenger.log 2>&1
This will allow any dependencies that may have been added to be installed automatically. Additionally, the application will be restarted with each deployment, ensuring that the most recent version of the code is currently running.
Miscellenious tips
Restart an application
To restart your Flask application manually, run the following command:
passenger-config restart-app /var/www/vhosts/flaskdemo.jmcouillard.com/httpdocs
View the logs
Phusion Passenger will display the following message if there is a problem:
To access the mentioned logs, follow the instructions in this article and execute a request to the application via the web browser.